Create an SNS Topic
What is an Amazon SNS topic?
It is a logical access point. It works as a form of communication channel. It allows you to group various endpoints. For example, SQS, Lambda, an email address or HTTP/S.
When do you Create an SNS Topic ?
The very first step to do in Amazon SNS is to get a topic created.
In this tutorial you will learn how to use the Management Console, the SDK for Java, and the SDK for .NET for creating a topic.
If you want to Create an SNS Topic with the Management Console, go through the below steps::
- Login to the Amazon SNS console.
- Do one of the following:
- In the case that not any of the topics have ever been created under your AWS account before, read the description of Amazon SNS on the home page.
- In the case that some of the topics were previously created using your AWS account, from navigation panel, click on the option Topics.
Create an SNS Topic – Topics
- For the section named Create topic, fill in a Topic name, such as MyFirstTopic.
Create an SNS Topic – Topic Name
- You can optionally open the Encryption section and go over the below steps.
Create an SNS Topic – Encryption
- Click on the option Enable encryption.
- Set the CMK, which is short for customer master key.
For each CMK type, the Description, Account, and CMK ARN are displayed.
Important
In the case that you were not the CMK owner, or in the case that you’re logging in with an account with no kms:ListAliases and kms:DescribeKey permissions, you won’t be able to view information about the CMK on the Amazon SNS console.
You should tell the owner of the CMK to give you the required permissions.
-
-
- By default, the AWS managed CMK will be set for SNS as (Default) alias/aws/sns.
-
Keep in mind
Remember the below:
– When you use the Publish action on a topic with SSE enabled for the very first time, KMS will create the managed CMK for SNS.
– When you utilize the Management Console for setting the managed CMK for SNS for a specific topic, KMS will create the managed CMK for SNS.
– For utilizing a custom CMK using your own AWS account, click on the option showing the field of Customer master key (CMK). Then, select the custom CMK out of the listed ones.
Keep in mind
You can check the needed instructions on how to create Asymmetric CMKs and Symmetric CMKs.
-
-
- For the sake of utilizing a custom CMK ARN using your own AWS account or using a different one, type it down in the field of Customer master key (CMK).
-
5. The topic owner is the one who is set by default to subscribe to or publish the topic. You can optionally configure more access permissions, by simply expanding the section for Access policy.
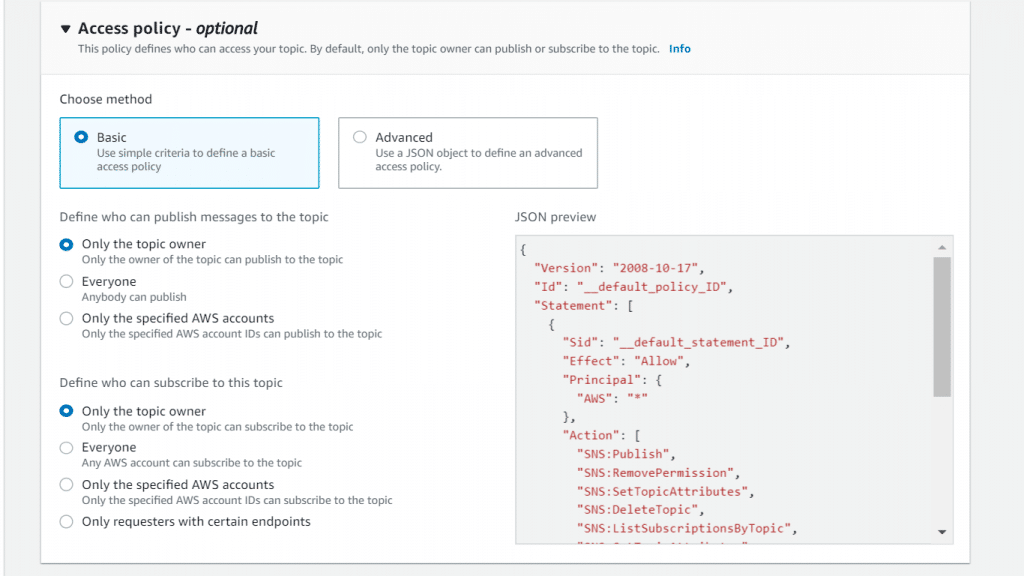
Create an SNS Topic – Access Policy
Keep in mind
Upon creating a topic through the console, you will find that the default policy utilizes the condition key of aws:SourceOwner. It is like the aws:SourceAccount.
6. You can optionally configure the way SNS tends to retry any failed attempts of message delivery. To do so, simply expand the section called Delivery retry policy (HTTP/S).
7. You can optionally configure the way SNS will start logging the delivery of messages to CloudWatch. To do so, simply expand the section named Delivery status logging.
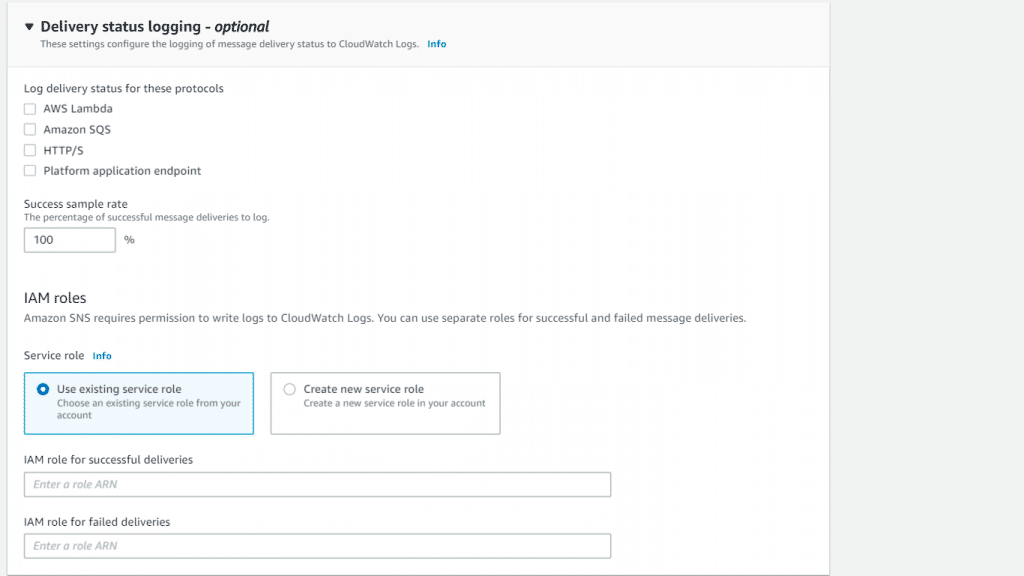
Create an SNS Topic – Delivery Status Logging
8. You can optionally start adding metadata tags to the topic by expanding the section called Tags. There you can go ahead and fill in a Key and a Value, then click on the option Add tag.
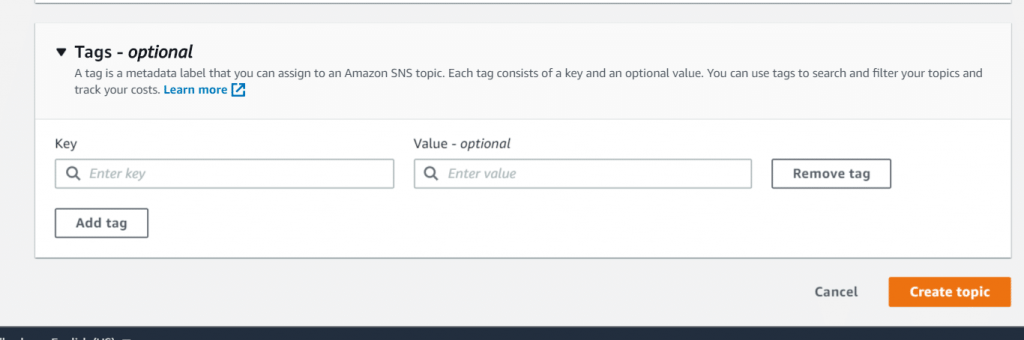
Create an SNS Topic – Add tags and Create
9. Click on the option Create topic.
The topic will get created and the page called MyFirstTopic will be displayed.
In the section called Details, you will find the topic’s Name, the ARN, its Display name, and the Topic owner‘s AWS account ID.
10. Finally, you will have to copy the topic ARN to the clipboard, which will look as follows:
arn:aws:sns:us-east-2:123456789012:MyFirstTopic
If you want to Create an SNS Topic through the AWS SDK for Java, go through the below steps::
- Setting your credentials.
- Typing your code.
Below you will find a code excerpt which is going to create the topic MyFirstTopic. After that it will print the topic ARN as well as the CreateTopicRequest request ID for a request which has been executed earlier and turned out to be successful.
// Here we are creating an SNS topic.
final CreateTopicRequest createTopicRequest = new CreateTopicRequest("MyFirstTopic");
final CreateTopicResponse createTopicResponse = snsClient.createTopic(createTopicRequest);
// Here we are printing the topic ARN.
System.out.println("TopicArn:" + createTopicResponse.getTopicArn());
// Here we are printing the request ID for the action of CreateTopicRequest.
System.out.println("CreateTopicRequest: " + snsClient.getCachedResponseMetadata(createTopicRequest));
3. Now, start compiling and running the code.
The topic will get created. Also, the topic ARN as well as the CreateTopicRequest request ID will get printed, such as follows:
TopicArn: arn:aws:sns:us-east-2:123456789012:MyFirstTopic
CreateTopicRequest: {AWS_REQUEST_ID=1234a567-bc89-012d-3e45-6fg7h890123i}
4. The topic ARN may be assigned to a String variable for utilizing it in extra operations, such as follows:
final String topicArn = "arn:aws:sns:us-east-2:123456789012:MyFirstTopic";
If you’d like to Create an SNS Topic with the AWS SDK for .NET, go through the below steps:
- Setting your AWS credentials.
- Typing in your code.
Below is a code excerpt which is going to Create an SNS Topic MyFirstTopic and later on print the topic ARN as well as the CreateTopicRequest request ID.
// Here we are going to Create an SNS Topic.
CreateTopicRequest createTopicRequest = new CreateTopicRequest("MyFirstTopic");
CreateTopicResponse createTopicResponse = snsClient.CreateTopic(createTopicRequest);
// Here we are printing the topic ARN.
Console.WriteLine("TopicArn: " + createTopicResponse.TopicArn);
// Here we are printing the request ID for the action of CreateTopicRequest.
Console.WriteLine("CreateTopicRequest: " + createTopicResponse.ResponseMetadata.RequestId);
3. Now, it’s time to get your code compiled and running.
The topic will become created and you will find that the topic ARN and CreateTopicRequest request ID will get printed, such as follows:
TopicArn: arn:aws:sns:us-east-2:123456789012:MyFirstTopic
CreateTopicRequest: 1234a567-bc89-012d-3e45-6fg7h890123i
4. It’s possible to get the topic ARN assigned to a String variable for utilizing it in more operations, such as:
String topicArn = createTopicResponse.TopicArn;