Manage Cluster Security Groups
In this article we will supply you with an example of how to manage cluster security groups. This article is complementary with the AWS SDK and Redshift article. You need to login with an EC2-Classic AWS account to be able to access the Amazon Redshift console for creating a cluster security group.
It shows the operations that are made to manage cluster security groups, such as:
- Getting a new cluster security group created.
Manage Cluster Security Groups – Create Cluster Security Group
- Getting ingress rules added to a cluster security group.
Manage Cluster Security Groups – Add Ingress Rules
- Getting a cluster security group associated with a cluster through the modification of cluster configuration.
Manage Cluster Security Groups – Modifying Cluster Configuration
Before learning how to manage cluster security groups, you must learn what they are:
A cluster security group is made up of a set of rules. Those rules are capable of controlling the access to a cluster. Individual rules specify a range of IP addresses or an EC2 security group which is granted access to the cluster. As soon as a cluster gets associated with a cluster security group, the set of rules specified in this cluster security group will be able to take control over the access to the cluster.
Redshift offers a “default” named cluster security group, created upon launching your very 1st cluster. It represents an empty cluster security group, which can get some inbound access rules added to it. Then, it is possible for you to associate this default cluster security group with your specified Redshift cluster.
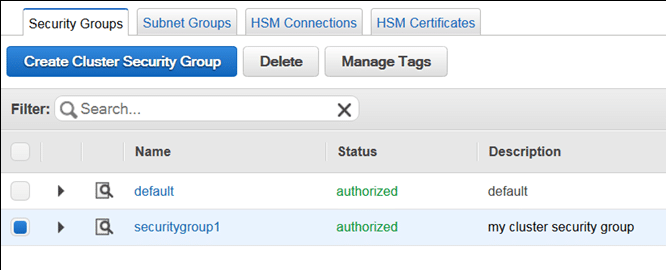
Manage Cluster Security Groups – Default Cluster Security Group
How to Manage Cluster Security Groups?
Upon creating a new cluster security group, by default it will not include any ingress rules. The following shown example will help you modify a new cluster security group through the addition of 2 ingress rules. 1 of the ingress rules gets added through the specification of a CIDR/IP range, while the 2nd ingress rule gets added through the specification of ID an EC2 security group combination and an owner ID.
To learn how you can start running the below example, see how to Run Java examples for Redshift with Eclipse. You must first update the code and supply a specific cluster identifier, as well as an account number.
/**
*
* The following file needs the Apache License; Version 2.0 called the "License".
* This file is not capable of being used without having the needed license. You can find a copy of
* this required License at the below link
*
* http://aws.amazon.com/apache2.0/
*
*/
// snippet-sourcedescription:[CreateAndModifyClusterSecurityGroup shows the way to get a Redshift security group created and modified.]
// snippet-service:[redshift]
// snippet-keyword:[Java]
// snippet-keyword:[Amazon Redshift]
// snippet-keyword:[Code Sample]
// snippet-keyword:[CreateClusterSecurityGroup]
// snippet-keyword:[DescribeClusterSecurityGroups]
// snippet-sourcetype:[full-example]
// snippet-sourcedate:[2019-02-01]
// snippet-sourceauthor:[AWS]
// snippet-start:[redshift.java.CreateAndModifyClusterSecurityGroup.complete]
package com.amazonaws.services.redshift;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import com.amazonaws.services.redshift.model.*;
public class CreateAndModifyClusterSecurityGroup {
public static AmazonRedshift client;
public static String clusterSecurityGroupName = "securitygroup1";
public static String clusterIdentifier = "***enter a specific cluster identifier***";
public static String ownerID = "***enter a 12-digit account number***";
public static void main(String[] args) throws IOException {
// A default client will be utilizing the {@link com.amazonaws.auth.DefaultAWSCredentialsProviderChain}
client = AmazonRedshiftClientBuilder.defaultClient();
try {
createClusterSecurityGroup();
describeClusterSecurityGroups();
addIngressRules();
associateSecurityGroupWithCluster();
} catch (Exception e) {
System.err.println("Operation failed: " + e.getMessage());
}
}
private static void createClusterSecurityGroup() {
CreateClusterSecurityGroupRequest request = new CreateClusterSecurityGroupRequest()
.withDescription("my cluster security group")
.withClusterSecurityGroupName(clusterSecurityGroupName);
client.createClusterSecurityGroup(request);
System.out.format("Created cluster security group: '%s'\n", clusterSecurityGroupName);
}
private static void addIngressRules() {
AuthorizeClusterSecurityGroupIngressRequest request = new AuthorizeClusterSecurityGroupIngressRequest()
.withClusterSecurityGroupName(clusterSecurityGroupName)
.withCIDRIP("192.168.40.5/32");
ClusterSecurityGroup result = client.authorizeClusterSecurityGroupIngress(request);
request = new AuthorizeClusterSecurityGroupIngressRequest()
.withClusterSecurityGroupName(clusterSecurityGroupName)
.withEC2SecurityGroupName("default")
.withEC2SecurityGroupOwnerId(ownerID);
result = client.authorizeClusterSecurityGroupIngress(request);
System.out.format("\nAdded ingress rules to security group '%s'\n", clusterSecurityGroupName);
printResultSecurityGroup(result);
}
private static void associateSecurityGroupWithCluster() {
// Here you will be getting existing security groups that are utilized by the cluster.
DescribeClustersRequest request = new DescribeClustersRequest()
.withClusterIdentifier(clusterIdentifier);
DescribeClustersResult result = client.describeClusters(request);
List<ClusterSecurityGroupMembership> membershipList =
result.getClusters().get(0).getClusterSecurityGroups();
List<String> secGroupNames = new ArrayList<String>();
for (ClusterSecurityGroupMembership mem : membershipList) {
secGroupNames.add(mem.getClusterSecurityGroupName());
}
// Here you will be adding new security group to the list.
secGroupNames.add(clusterSecurityGroupName);
// Here you will be applying the change to the cluster.
ModifyClusterRequest request2 = new ModifyClusterRequest()
.withClusterIdentifier(clusterIdentifier)
.withClusterSecurityGroups(secGroupNames);
Cluster result2 = client.modifyCluster(request2);
System.out.format("\nAssociated security group '%s' to cluster '%s'.", clusterSecurityGroupName, clusterIdentifier);
}
private static void describeClusterSecurityGroups() {
DescribeClusterSecurityGroupsRequest request = new DescribeClusterSecurityGroupsRequest();
DescribeClusterSecurityGroupsResult result = client.describeClusterSecurityGroups(request);
printResultSecurityGroups(result.getClusterSecurityGroups());
}
private static void printResultSecurityGroups(List<ClusterSecurityGroup> groups)
{
if (groups == null)
{
System.out.println("\nDescribe cluster security groups result is null.");
return;
}
System.out.println("\nPrinting security group results:");
for (ClusterSecurityGroup group : groups)
{
printResultSecurityGroup(group);
}
}
private static void printResultSecurityGroup(ClusterSecurityGroup group) {
System.out.format("\nName: '%s', Description: '%s'\n", group.getClusterSecurityGroupName(), group.getDescription());
for (EC2SecurityGroup g : group.getEC2SecurityGroups()) {
System.out.format("EC2group: '%s', '%s', '%s'\n", g.getEC2SecurityGroupName(), g.getEC2SecurityGroupOwnerId(), g.getStatus());
}
for (IPRange range : group.getIPRanges()) {
System.out.format("IPRanges: '%s', '%s'\n", range.getCIDRIP(), range.getStatus());
}
}
}
// snippet-end:[redshift.java.CreateAndModifyClusterSecurityGroup.complete]
The code example above is a necessary step for the AWS SDK and Redshift src directory file for your project. It is in order to make sure that the src directory file is: CreateAndModifyClusterSecurityGroup.java