AWS SDK and Redshift
The AWS SDK and Redshift may be used together for building clients. The SDK for Java offers a class with the name AmazonRedshiftClientBuilder for interacting with Redshift.
Keep in Mind
Using the SDK for Java you can get thread-safe clients in order to access Redshift. The best thing to do is to create only 1 client with your application, then keep reusing this same client between multiple threads.
Both the AwsClientBuilder and the AmazonRedshiftClientBuilder classes for the sake of configuring an endpoint or creating an AmazonRedshift client.
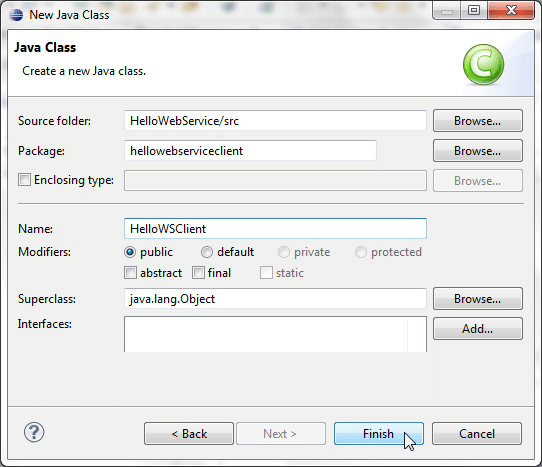
AWS SDK and Redshift – Java Client Class
After that, the client object could be utilized in order to get a Cluster object instance created. This cluster object includes methods capable of mapping to underlying actions of Redshift Query API.
A request object needs to be created in correspondence to calling a method. The request object contains data which is needed to get passed with the request.
The Cluster object offers data that returns from Redshift as a response to the actual request.
In the below example you will see how the AmazonRedshiftClientBuilder class can be utilized for the sake of configuring an endpoint, then get a 2-node ds2.xlarge cluster created.
String endpoint = "https://redshift.us-east-1.amazonaws.com/";
String region = "us-east-1";
AwsClientBuilder.EndpointConfiguration config = new AwsClientBuilder.EndpointConfiguration(endpoint, region);
AmazonRedshiftClientBuilder clientBuilder = AmazonRedshiftClientBuilder.standard();
clientBuilder.setEndpointConfiguration(config);
AmazonRedshift client = clientBuilder.build();
CreateClusterRequest request = new CreateClusterRequest()
.withClusterIdentifier("exampleclusterusingjava")
.withMasterUsername("masteruser")
.withMasterUserPassword("12345678Aa")
.withNodeType("ds2.xlarge")
.withNumberOfNodes(2);
Cluster createResponse = client.createCluster(request);
System.out.println("Created cluster " + createResponse.getClusterIdentifier());
Run Java examples for AWS SDK and Redshift with Eclipse:
General process of running Java code examples using Eclipse
- First you need to get a new AWS Java Project created in Eclipse.
AWS SDK and Redshift – New AWS Java Project
Go over the procedure in AWS Eclipse Toolkit Setup for downloading and setting up your AWS Toolkit for Eclipse.
- Get the sample code copied from this document’s section which you are reading. Then, go ahead and paste it to your project as a new Java class file.
- Stat running the code.
Run Java examples from command line for AWS SDK and Redshift:
How to run Java code examples using command line for AWS SDK and Redshift?
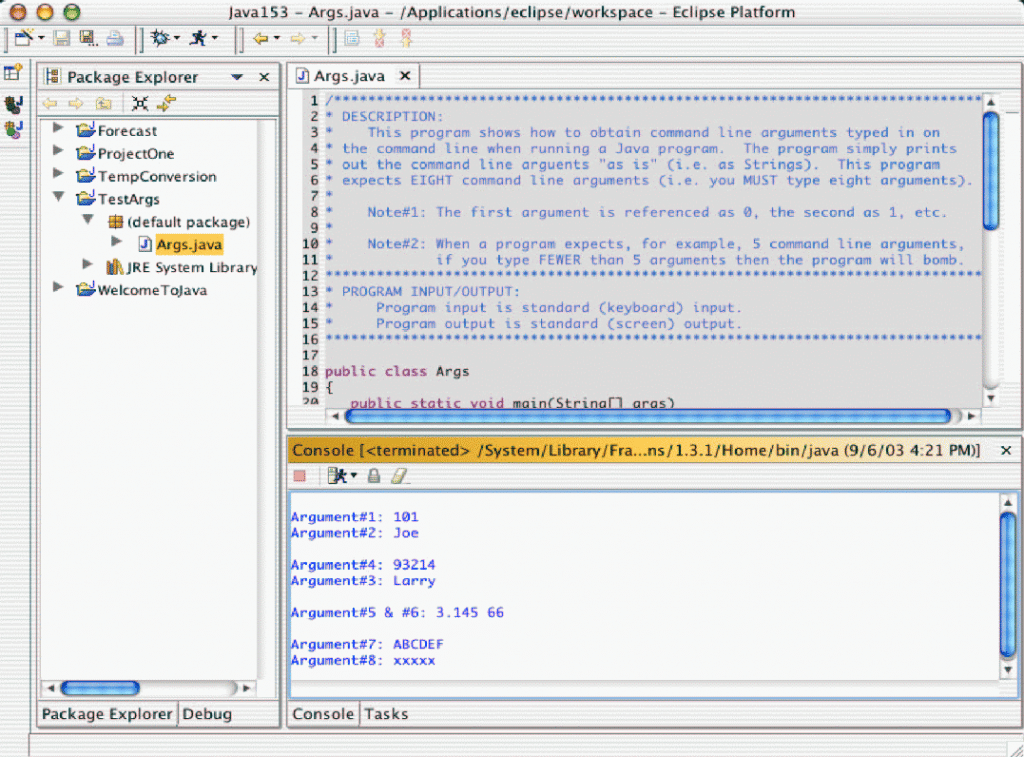
AWS SDK and Redshift – Running Java Code Examples
- First follow the below steps for setting up and testing your environment:
- After creating a directory for working, create src in it. Also create sdk subfolders and bin.
- Begin downloading the SDK for Java then start unzipping it to your created sdk subfolder. When the SDK is unzipped, you will find 4 subdirectories in your sdk folder. Included with them is a third-party folder and a lib.
- Enter your credentials to the SDK.
- Make sure of being able to run javac and java using the working directory you have. They can be tested when you run the below 2 commands:
javac -help
java -help
- Copy your code to a .java file, then save it inside the src folder. For going over the necessary steps, you will have to utilize the code shown in Manage Cluster Security Groups in order to ensure that the src directory file is: CreateAndModifyClusterSecurityGroup.java.
- Start compiling your code.
javac -cp sdk/lib/aws-java-sdk-1.3.18.jar -d bin src\CreateAndModifyClusterSecurityGroup.java
In case of utilizing another SDK version for Java, you will need to change the classpath (-cp) to suit that version.
- Start running your code. The below commands include line breaks that are used for improving readability.
java -cp "bin;
sdk/lib/*;
sdk/third-party/commons-logging-1.1.1/*;
sdk/third-party/httpcomponents-client-4.1.1/*;
sdk/third-party/jackson-core-1.8/*"
CreateAndModifyClusterSecurityGroup
Make alterations to the used class path separator based on what is required for the operating system you’re working with. Such as using the “;” separator for a Windows OS like the one in the example, or the “:” separator for a Unix OS. Different coding could need additional libraries than the ones found in the above shown example. Also, the SDK version you are utilizing could include other 3rd party names for your folder than the used above. When this occurs, you will need to change the classpath (-cp) owever required.
For the sake of running some samples in the following document, you will need to utilize an SDK version supporting Redshift. For receiving the newest version of SDK for Java, check out the downloadable AWS SDK for Java.
How to set the client endpoint for AWS SDK and Redshift?
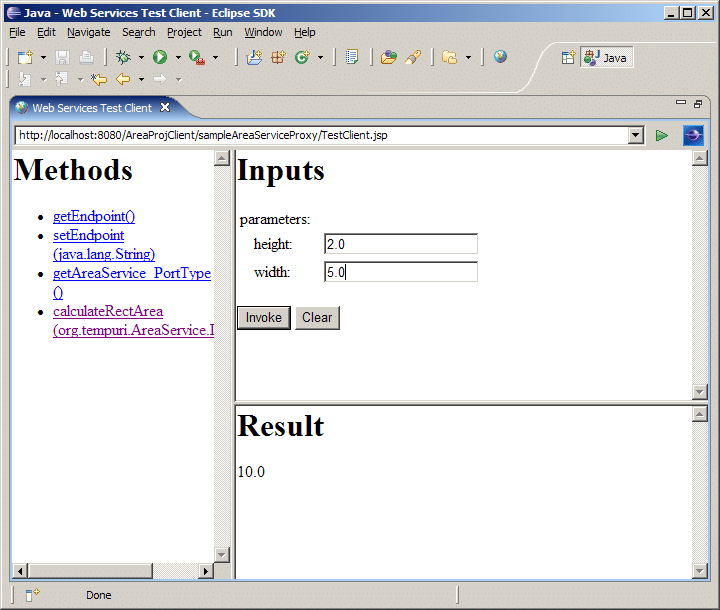
AWS SDK and Redshift – Setting Client Endpoints
The endpoint https://redshift.us-east-1.amazonaws.com/ is utilized by the SDK for Java. It can be set with the client.setEndpoint method. Below you can see an example:
client = new AmazonRedshiftClient(credentials);
client.setEndpoint("https://redshift.us-east-1.amazonaws.com/");