AWS SDK Metrics
The AWS SDK for Java can generate AWS SDK Metrics are generated for the sake of gaining better visualization. It also allows you to perform monitoring using CloudWatch for measuring:
- App performance when accessing AWS
- JVMs performance when used with AWS
- Details for runtime environment. For example: number of threads, opened file descriptors and heap memory.
Keep in mind
You can also use the SDK Metrics for Enterprise Support in order to get your application’s metrics. SDK Metrics is a service offered by AWS for publishing data to CloudWatch. It permits you to get metric data shared with Support for a more efficient and simplified troubleshooting process.
Enabling AWS SDK Metric Generation:
AWS SDK metrics will be disabled by default. For the sake of enabling it for your environment, you need to add a system property which show where your security credential file is upon the JVM startup. Just like the below example:
-Dcom.amazonaws.sdk.enableDefaultMetrics=credentialFile=/path/aws.properties
Declare your credential file’s path in order to get the found datapoints uploaded to CloudWatch to analyze them.
Keep in mind
In case you want to access AWS using an EC2 instance from EC2 instance metadata service, it’s not required of you to set a credential file. For this, you will merely have to set the following:
-Dcom.amazonaws.sdk.enableDefaultMetrics
Every captured metric using the SDK will be found below namespace AWSSDK/Java, then they get uploaded to the default region for CloudWatch, which is us-east-1. For the sake of altering regions, you can set them with the cloudwatchRegion attribute. Utilize the below statement for setting your CloudWatch region to us-west-2:
-Dcom.amazonaws.sdk.enableDefaultMetrics=credentialFile=/path/aws.properties,cloudwatchRegion=us-west-2
Upon enabling the feature, metric data points are going to be generated whenever there occurs a service request to AWS from the SDK. Also they get queued for statistical summary, as well as uploaded in an asynchronous manner to CloudWatch each minute. Upon uploading those metrics, they can be visualized through AWS Management Console. You can also get alarms set on possible errors like leakage of file descriptor or memory. To know more about Cloudwatch alarms you can check the EC2 Instances: Status Check Alarms guidline.
How to get AWS SDK Metrics from CloudWatch?
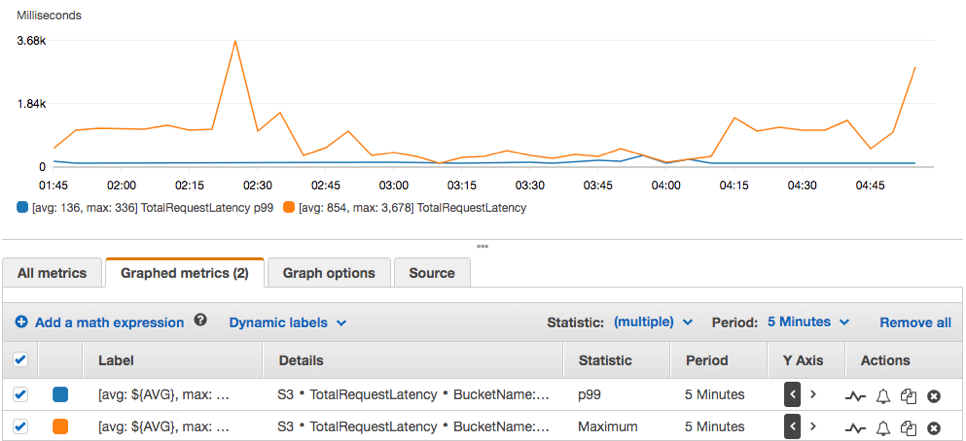
AWS SDK Metrics – AWS SDK Metrics from CloudWatch
Ways for listing AWS SDK Metrics:
For the sake of listing CloudWatch metrics, you will need to get a ListMetricsRequest created. After that, the AmazonCloudWatchClient’s listMetrics method should be called. The ListMetricsRequest may be used for the sake of filtering returned metrics based on metric names, dimensions or namespace.
Keep in mind
A list of available dimensions and AWS SDK metrics can be found posted by services.
The Imports needed
import com.amazonaws.services.cloudwatch.AmazonCloudWatch;import com.amazonaws.services.cloudwatch.AmazonCloudWatchClientBuilder;import com.amazonaws.services.cloudwatch.model.ListMetricsRequest;import com.amazonaws.services.cloudwatch.model.ListMetricsResult;import com.amazonaws.services.cloudwatch.model.Metric;
The Coding
final AmazonCloudWatch cw = AmazonCloudWatchClientBuilder.defaultClient(); ListMetricsRequest request = new ListMetricsRequest() .withMetricName(name) .withNamespace(namespace); boolean done = false; while(!done) { ListMetricsResult response = cw.listMetrics(request); for(Metric metric : response.getMetrics()) { System.out.printf( “Retrieved metric %s”, metric.getMetricName()); } request.setNextToken(response.getNextToken()); if(response.getNextToken() == null) { done = true; }}
When the getMetrics method is called, you will get the metrics returned in a ListMetricsResult.
Results can get paged.
In order to get the following batch of results, the setNextToken needs to get called on the original request object. It’s return value should be the ListMetricsResult object’s getNextToken method. The modified request object should be passed to a different call to listMetrics.
Available AWS SDK Metrics Types:
There is a default set of AWS SDK metrics separated into 3 main types.
What are Request AWS SDK Metrics?
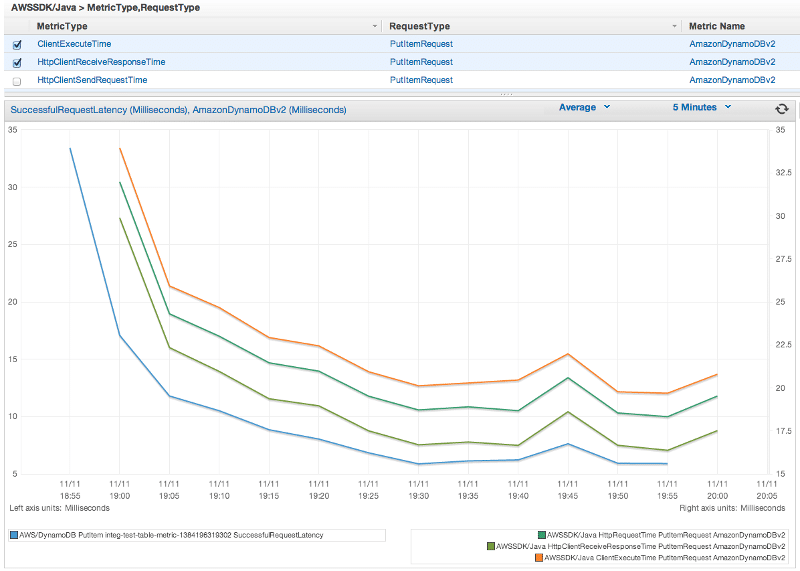
AWS SDK Metrics – Request AWS SDK Metrics
They are the metrics capable of covering areas like:
– The number of requests
– The retries
– The latency of the HTTP request and response
– The exceptions
What are Service AWS SDK Metrics?
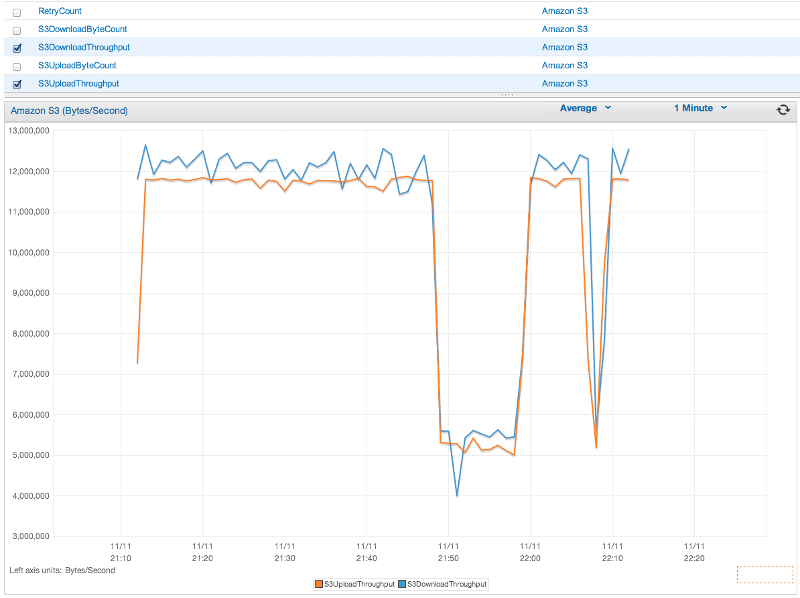
AWS SDK Metrics – Service AWS SDK Metrics
They are the metrics that include service-specific data. For example, the byte count and the throughput of S3 downloads and uploads.
What are Machine AWS SDK Metrics?
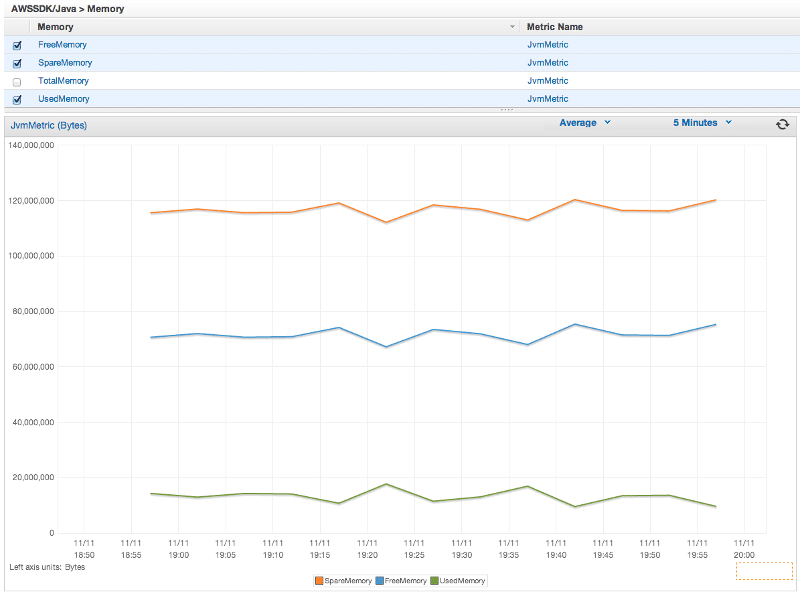
AWS SDK Metrics – Machine AWS SDK Metrics
They are the metrics capable of covering the runtime environment. As well as heap memory, the open file descriptions, and the amount of threads.
For not including Machine Metrics, you can add the parameter of excludeMachineMetrics to your system property as follows:
-Dcom.amazonaws.sdk.enableDefaultMetrics=credentialFile=/path/aws.properties,excludeMachineMetrics